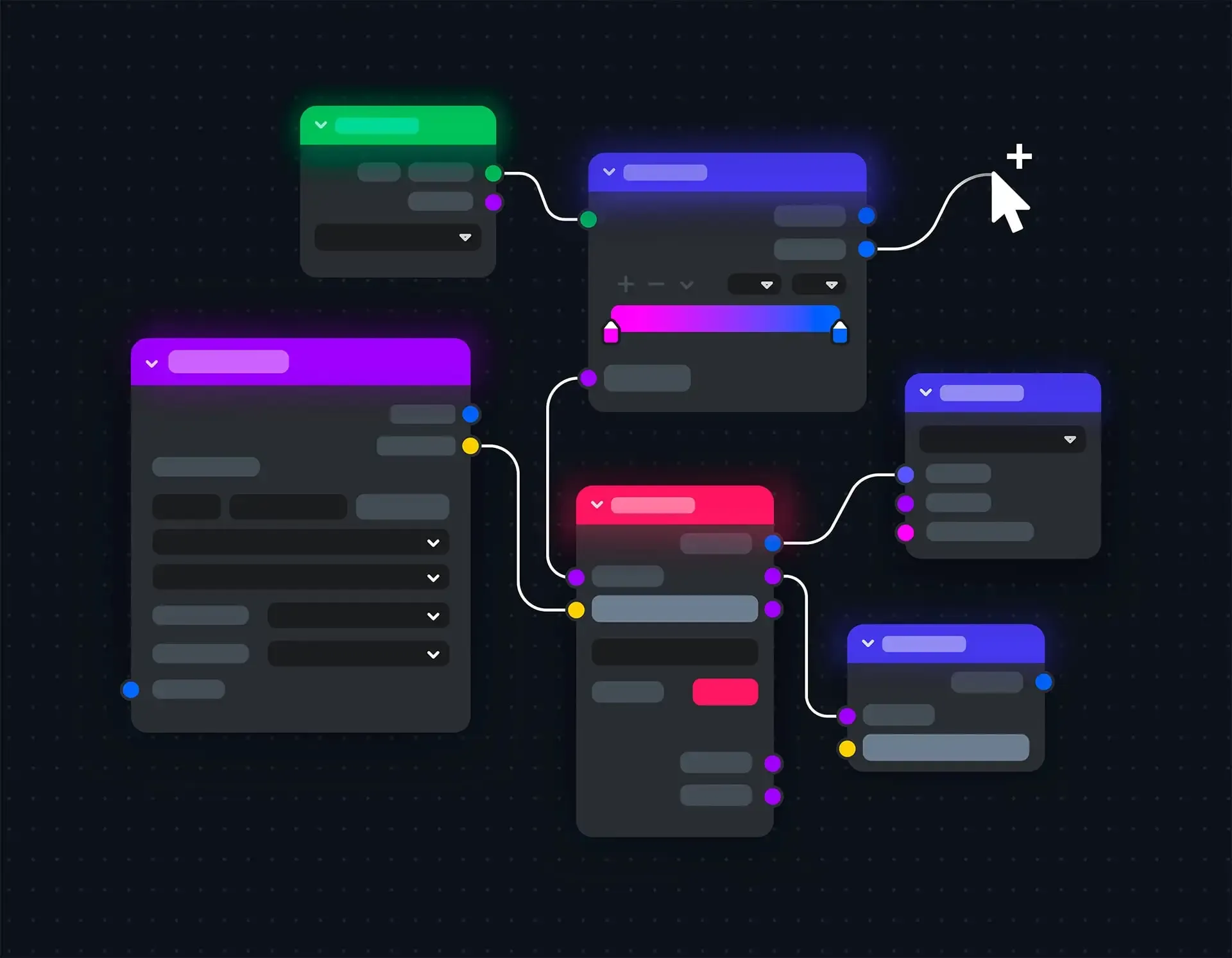
Introduction
Have you ever inherited a CSS codebase that made you want to start from scratch? We’ve all been there: specificity wars, duplicate code, inconsistent naming conventions, and the dreaded “!important” flags scattered throughout thousands of lines of styles. In enterprise applications, these problems don’t just cause developer headaches—they result in inconsistent user experiences, slower performance, and significantly higher maintenance costs. As applications grow in complexity and team sizes increase, the need for a structured, scalable CSS architecture becomes not just beneficial but essential.
In this article, we’ll explore proven methodologies for organizing CSS in large-scale enterprise applications. You’ll learn how to implement a scalable architecture that can support dozens of developers working simultaneously while maintaining consistency, reducing CSS bloat, and improving performance. Whether you’re building a new enterprise application or refactoring an existing one, these principles and practical examples will help you establish a CSS architecture that can grow with your project.
Understanding CSS architecture challenges
Before diving into solutions, it’s important to understand the unique challenges that enterprise applications face when it comes to CSS. Unlike smaller websites or applications, enterprise projects typically involve multiple teams working concurrently, legacy code considerations, strict performance requirements, and the need to maintain visual consistency across hundreds of components.
The primary challenges include specificity management, avoiding style conflicts, maintaining consistency across large codebases, and ensuring performant delivery of styles. In most enterprise environments, we’ve seen CSS files grow to hundreds of kilobytes or even megabytes, with complex dependency chains and specificity issues that make maintenance nearly impossible. One financial services client we worked with had accumulated over 15,000 lines of CSS with numerous specificity conflicts, resulting in inconsistent components and significant performance issues on mobile devices.
To address these challenges effectively, we need a systematic approach that encompasses organization, methodology, and technical implementation. Let’s explore each of these aspects in detail.
Architectural methodologies for enterprise CSS
When it comes to CSS methodologies for large-scale applications, several approaches have proven effective. Rather than advocating for a single “correct” methodology, we’ll examine the strengths of different approaches and how they can be combined for optimal results.
BEM and namespacing
BEM (Block, Element, Modifier) provides a naming convention that helps create self-contained components:
/* Block component */
.card {}
/* Element that depends on the block */
.card__title {}
/* Modifier that changes the style of the block */
.card--featured {}
For enterprise applications, we recommend extending BEM with namespaces to further organize components by their purpose:
/* Component namespace */
.c-card {}
.c-card__title {}
/* Utility namespace */
.u-visually-hidden {}
/* Layout namespace */
.l-grid {}
/* Theme namespace */
.t-dark {}
This namespacing approach immediately communicates the purpose and scope of each class, making large codebases more navigable.
CUBE CSS
For the most complex enterprise applications, we’ve found that the CUBE CSS methodology (Composition, Utility, Block, Exception) offers an excellent balance of flexibility and structure. This approach combines the best aspects of utility-first CSS with the modularity of BEM:
/* Composition layer */
.l-cluster {
display: flex;
flex-wrap: wrap;
gap: var(--space-s);
}
/* Utility layer */
.u-visually-hidden {
position: absolute;
width: 1px;
height: 1px;
overflow: hidden;
clip: rect(0 0 0 0);
}
/* Block layer */
.c-card {
padding: var(--space-m);
border-radius: var(--border-radius-m);
background-color: var(--color-surface);
}
/* Exception */
.c-card.is-featured {
background-color: var(--color-accent);
}
The layered approach of CUBE CSS helps manage complexity by clearly separating concerns and creating a predictable cascade.
Folder structure and organization
A well-organized folder structure is critical for maintenance and scalability. Here’s a folder structure we’ve implemented successfully across multiple enterprise projects:
styles/
├── settings/ # Variables, tokens, config
│ ├── colors.css
│ ├── typography.css
│ ├── spacing.css
│ └── breakpoints.css
├── tools/ # Mixins and functions
│ ├── media-queries.css
│ └── typography.css
├── global/ # Reset and base styles
│ ├── reset.css
│ └── typography.css
├── layouts/ # Layout components
│ ├── grid.css
│ └── cluster.css
├── components/ # UI components
│ ├── button/
│ │ ├── button.css
│ │ └── button.variants.css
│ ├── card/
│ │ ├── card.css
│ │ └── card.variants.css
│ └── modal/
│ ├── modal.css
│ └── modal.js
├── utilities/ # Utility classes
│ ├── spacing.css
│ ├── typography.css
│ └── visibility.css
└── themes/ # Theme variations
├── light.css
└── dark.css
This separation creates clear boundaries between different types of styles and makes it easier for teams to work in parallel without conflicts. Component styles are isolated in their own directories, often with accompanying JavaScript if needed.
For enterprise applications, we recommend a component-first approach where each UI component has its own directory containing all related styles, variants, documentation, and tests:
components/
└── button/
├── button.css # Core styles
├── button.variants.css # Variations
├── button.test.js # Component tests
├── button.stories.js # Storybook documentation
└── button.usage.md # Usage guidelines
This structure facilitates a truly modular approach where components can be developed, tested, and documented in isolation.
Design tokens and CSS custom properties
Design tokens form the foundation of a scalable CSS architecture by creating a single source of truth for visual design elements. In enterprise applications, implementing a robust design token system is essential for consistency and maintenance:
/* settings/tokens.css */
:root {
/* Colors */
--color-primary-100: #EBF5FF;
--color-primary-500: #0066FF;
--color-primary-900: #003399;
/* Semantic colors */
--color-background: var(--color-primary-100);
--color-text: #333333;
--color-accent: var(--color-primary-500);
/* Spacing */
--space-xs: 0.25rem;
--space-s: 0.5rem;
--space-m: 1rem;
--space-l: 1.5rem;
--space-xl: 2rem;
/* Typography */
--font-family-base: 'Inter', sans-serif;
--font-size-s: 0.875rem;
--font-size-m: 1rem;
--font-size-l: 1.25rem;
--font-size-xl: 1.5rem;
--font-size-xxl: 2rem;
}
By using CSS custom properties (variables) for all design tokens, we create a flexible system that can support theming, component variations, and responsive adaptations:
/* components/card/card.css */
.c-card {
padding: var(--space-m);
background-color: var(--color-surface);
border-radius: var(--border-radius-m);
box-shadow: var(--shadow-s);
}
/* themes/dark.css */
.t-dark {
--color-background: #121212;
--color-surface: #242424;
--color-text: #FFFFFF;
}
This token-based approach provides a foundation for both consistency and flexibility.
Building component systems
Enterprise applications typically contain hundreds of UI components, making a systematic approach to component development essential. We recommend developing components using these principles:
- Component isolation: Each component should be self-contained with minimal external dependencies.
- Progressive enhancement: Core functionality should work without JavaScript where possible.
- Responsive behavior: Components should adapt to both viewport and container contexts.
- Accessibility: Built-in from the start, not added later.
Here’s an example of a well-structured card component:
/* components/card/card.css */
.c-card {
--card-padding: var(--space-m);
--card-border-radius: var(--border-radius-m);
display: flex;
flex-direction: column;
padding: var(--card-padding);
border-radius: var(--card-border-radius);
background-color: var(--color-surface);
box-shadow: var(--shadow-s);
}
.c-card__header {
display: flex;
align-items: center;
margin-bottom: var(--space-s);
}
.c-card__title {
font-size: var(--font-size-l);
font-weight: var(--font-weight-bold);
color: var(--color-text);
margin: 0;
}
.c-card__content {
flex: 1;
}
.c-card__footer {
margin-top: var(--space-m);
display: flex;
justify-content: flex-end;
gap: var(--space-s);
}
/* Component variants */
.c-card--featured {
background-color: var(--color-accent);
color: white;
box-shadow: var(--shadow-m);
}
.c-card--compact {
--card-padding: var(--space-s);
}
This approach uses CSS custom properties to create component-specific variations while maintaining the connection to global design tokens.
Performance optimization
CSS performance is a critical concern for enterprise applications, particularly for global user bases with varying network conditions. Here are key strategies we implement:
Critical CSS
Extract and inline critical styles for the initial viewport:
<head>
<style>
/* Critical styles for above-the-fold content */
.c-header, .c-hero { /* ... */ }
</style>
<link rel="stylesheet" href="main.css" media="print" onload="this.media='all'">
</head>
Code splitting
Separate CSS by route or feature to avoid loading unnecessary styles:
// In a React application using CSS Modules
import styles from './Dashboard.module.css';
// In a build system like Webpack
import './pages/dashboard.css';
Reducing specificity
Keep selectors simple to improve both maintenance and performance:
/* Avoid */
.sidebar .sidebar-nav ul li a.active { /* ... */ }
/* Prefer */
.c-nav__link--active { /* ... */ }
Responsible use of utilities
Utility classes can reduce overall CSS size when used strategically:
/* utilities/spacing.css */
.u-mt-s { margin-top: var(--space-s); }
.u-mt-m { margin-top: var(--space-m); }
.u-mt-l { margin-top: var(--space-l); }
Living style guide integration
Every enterprise CSS architecture should include a living style guide or design system documentation. This integration ensures that the CSS implementation stays aligned with design requirements and provides a reference for developers.
We typically build style guides using tools like Storybook, which allows components to be viewed, tested, and documented in isolation:
// components/button/button.stories.js
export default {
title: 'Components/Button',
component: Button,
argTypes: {
variant: {
options: ['primary', 'secondary', 'tertiary'],
control: { type: 'select' }
},
size: {
options: ['small', 'medium', 'large'],
control: { type: 'radio' }
}
}
};
const Template = (args) => <Button {...args} />;
export const Primary = Template.bind({});
Primary.args = {
variant: 'primary',
label: 'Button',
};
The style guide should document not just how components look, but how they should be used, their variants, and any implementation details that other developers need to know.
Try it yourself: implementing scalable CSS architecture
Ready to improve your own enterprise CSS architecture? Here’s a step-by-step approach:
-
Audit Your Current CSS
- Identify duplicate styles
- Find specificity issues
- Catalog inconsistent patterns
-
Establish Design Tokens
- Convert hard-coded values to variables
- Create a token naming system
- Document semantic usage
-
Choose a Methodology
- Select BEM, CUBE CSS, or a hybrid approach
- Document naming conventions
- Create examples for team reference
-
Implement Folder Structure
- Set up the directory organization
- Move files to appropriate locations
- Document the structure
-
Convert Key Components
- Start with high-visibility components
- Follow the new methodology
- Document in a style guide
-
Measure and Optimize
- Track CSS file size
- Monitor rendering performance
- Implement critical CSS
Conclusion
Building a scalable CSS architecture for enterprise applications is both art and science. It requires thoughtful organization, consistent methodology, and careful performance optimization. The approaches outlined in this article—using namespaced components, implementing design tokens with CSS custom properties, adopting methodologies like CUBE CSS, and creating a structured folder organization—provide a foundation that can scale with your application and team.
Remember that CSS architecture is not a one-time implementation but an ongoing process. As your application evolves, so should your CSS architecture. Regular refactoring, performance monitoring, and style guide maintenance will ensure that your CSS remains manageable, performant, and consistent.
For next steps, consider:
- Conducting an audit of your current CSS architecture
- Implementing a design token system
- Creating a simple style guide for your most-used components
- Setting up CSS performance monitoring
For further reading: