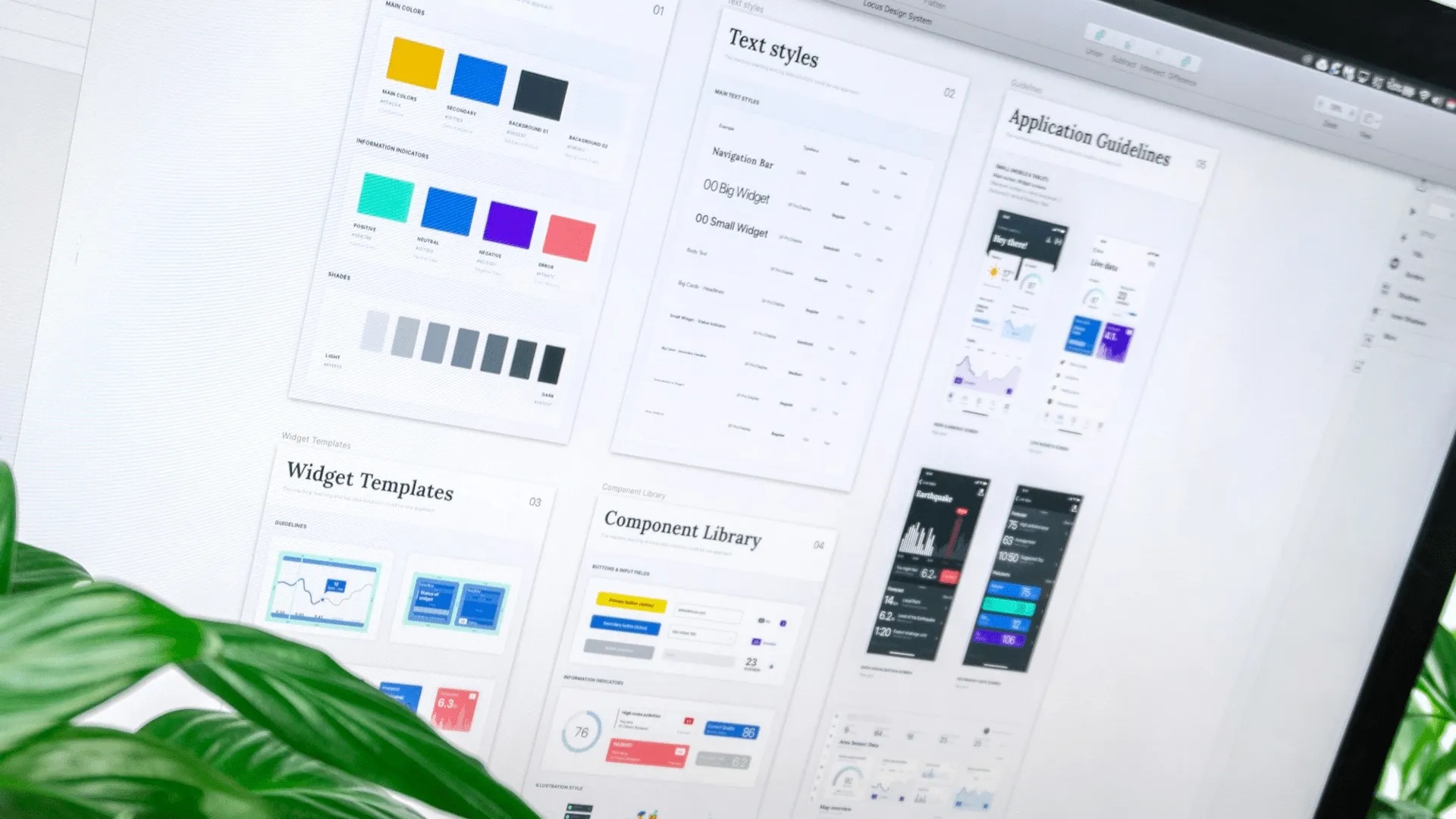
Introduction
Remember when we used to build components that could only respond to the viewport size? Those days are behind us. In 2025, design systems face a new challenge: creating components that are truly context-aware and self-contained. With the widespread adoption of container and style queries, we’re witnessing a paradigm shift in how we build responsive design systems. This isn’t just about making things look good at different screen sizes anymore—it’s about creating components that intelligently adapt to their immediate context, regardless of where they’re placed in the application.
In this article, we’ll explore how container queries and style queries are revolutionizing design systems, and provide practical examples of implementing these features in your projects. Whether you’re building a new design system or upgrading an existing one, understanding these concepts is crucial for creating future-proof components.
The evolution of responsive design
The journey from media queries to container queries represents a fundamental shift in how we approach responsive design. Media queries, while revolutionary when introduced, have a significant limitation: they can only respond to viewport characteristics. This creates challenges when the same component needs to behave differently based on its container rather than the screen size.
Consider a card component that appears in both a sidebar and the main content area. With media queries alone, you’d need to create specific overrides for each context, leading to tightly coupled components and maintenance headaches. Container queries solve this by allowing components to adapt based on their immediate container’s size.
@container (min-width: 400px) {
.card {
display: grid;
grid-template-columns: 200px 1fr;
gap: 1rem;
}
}
@container (max-width: 399px) {
.card {
display: flex;
flex-direction: column;
}
}
Understanding container queries
Container queries work by defining a containment context and then allowing child elements to query the dimensions of that container. Here’s how to implement them:
- First, establish a containment context:
.container {
container-type: inline-size;
container-name: card-container;
}
- Then, write queries that respond to that container:
@container card-container (min-width: 700px) {
.component {
/* Styles for larger containers */
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
}
}
This approach allows the same component to adapt differently when placed in different contexts, without knowing anything about the viewport size.
Style queries: the next evolution
Style queries take the concept further by allowing components to respond to custom properties set on their containers. This is particularly powerful for theme switching and dynamic styling based on context.
@container style(--theme-variant: professional) {
.component {
/* Professional theme styles */
border-radius: 2px;
box-shadow: var(--shadow-md);
}
}
@container style(--theme-variant: playful) {
.component {
/* Playful theme styles */
border-radius: 12px;
box-shadow: var(--shadow-lg);
}
}
Practical implementation in design systems
When implementing these features in your design system, consider the following structure:
- Base component definition:
.component {
/* Base styles */
container-type: inline-size;
container-name: component;
}
- Container-based responsive styles:
@container component (min-width: 300px) {
.component__element {
/* Larger container styles */
}
}
- Style query variations:
@container style(--component-density: compact) {
.component {
/* Compact styles */
}
}
Best practices and considerations
When implementing container and style queries in your design system, keep these best practices in mind:
-
Component isolation: Each component should be self-contained and not rely on parent dimensions unless explicitly needed.
-
Performance optimization: While container queries are efficient, excessive nesting of queried containers can impact performance. Keep your containment contexts meaningful and purposeful.
-
Progressive enhancement: Always provide sensible defaults for browsers that might not support these features:
/* Base styles for all browsers */
.component {
display: flex;
flex-direction: column;
}
/* Enhanced layout for container query support */
@supports (container-type: inline-size) {
.component {
container-type: inline-size;
}
@container (min-width: 400px) {
.component {
flex-direction: row;
}
}
}
Conclusion
Container queries and style queries represent a significant leap forward in how we build responsive design systems. They enable truly modular, context-aware components that can adapt to their surroundings without external dependencies. As we move forward in 2025, these features are becoming essential tools in every frontend developer’s toolkit.
To get started with implementing these features in your design system:
- Audit your existing components for viewport-dependent styles
- Identify components that would benefit from container-based responsiveness
- Gradually refactor your components to use container and style queries
- Update your documentation to reflect new patterns and best practices
For further reading: